Помогите новичку с игрой в Java
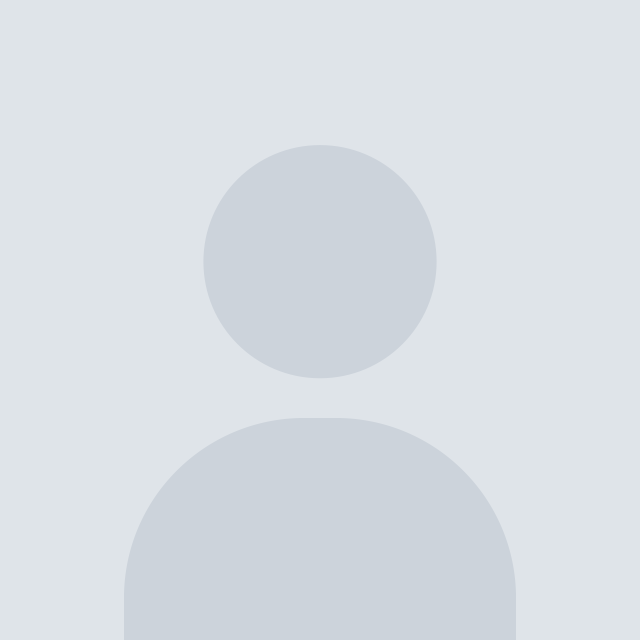
Помогите, пожалуйста, с одной проблемой. Как в начале игры Flappy Bird вывести картинку "start.png", после нажатия пробела должен появится обратный отсчёт 3...2...1 (картинки "3", "2", "1", которые меняются через 1 секунду) и после этого начнется сама игра, уже три дня над этим вопросом сижу, буду благодарна за помощь)
Ниже класс, в котором описываются основные методы и функции:
package com.mygdx.game;
import com.badlogic.gdx.ApplicationAdapter;
import com.badlogic.gdx.Gdx;
import com.badlogic.gdx.Input;
import com.badlogic.gdx.graphics.GL20;
import com.badlogic.gdx.graphics.Texture;
import com.badlogic.gdx.graphics.g2d.SpriteBatch;
public class MyGdxGame extends ApplicationAdapter {
SpriteBatch batch;
Background bg;
Bird bird;
Obstacles obstacles;
boolean gameOver;
Texture restartTexture;
public static final String title = "Flappy Bird";
@Override
public void create () {
batch = new SpriteBatch();
bg = new Background();
bird = new Bird();
obstacles = new Obstacles();
gameOver = false;
restartTexture = new Texture("restartBtn.png");
}
@Override
public void render () {
update();
batch.begin();
bg.render(batch);
obstacles.render(batch);
if(!gameOver) {
bird.render(batch);
}else{
batch.draw(restartTexture, 100, 250);
}
batch.end();
}
public void update(){
bg.update();
bird.update();
obstacles.update();
for (int i = 0; i < Obstacles.obs.length; i++) {
if(bird.position.x > Obstacles.obs[i].position.x && bird.position.x < Obstacles.obs[i].position.x+50){
if(!Obstacles.obs[i].emptySpace.contains(bird.position)){
gameOver = true;
}
}
}
if(bird.position.y <0 || bird.position.y > 600){
gameOver = true;
}
if(Gdx.input.isKeyPressed(Input.Keys.SPACE) && gameOver){
recreate();
}
}
@Override
public void dispose () {
batch.dispose();
}
public void recreate(){
bird.recreate();
obstacles.recreate();
gameOver = false;
}
}